1. coRouter 로 웹 요청 받기
1.1. Project 생성
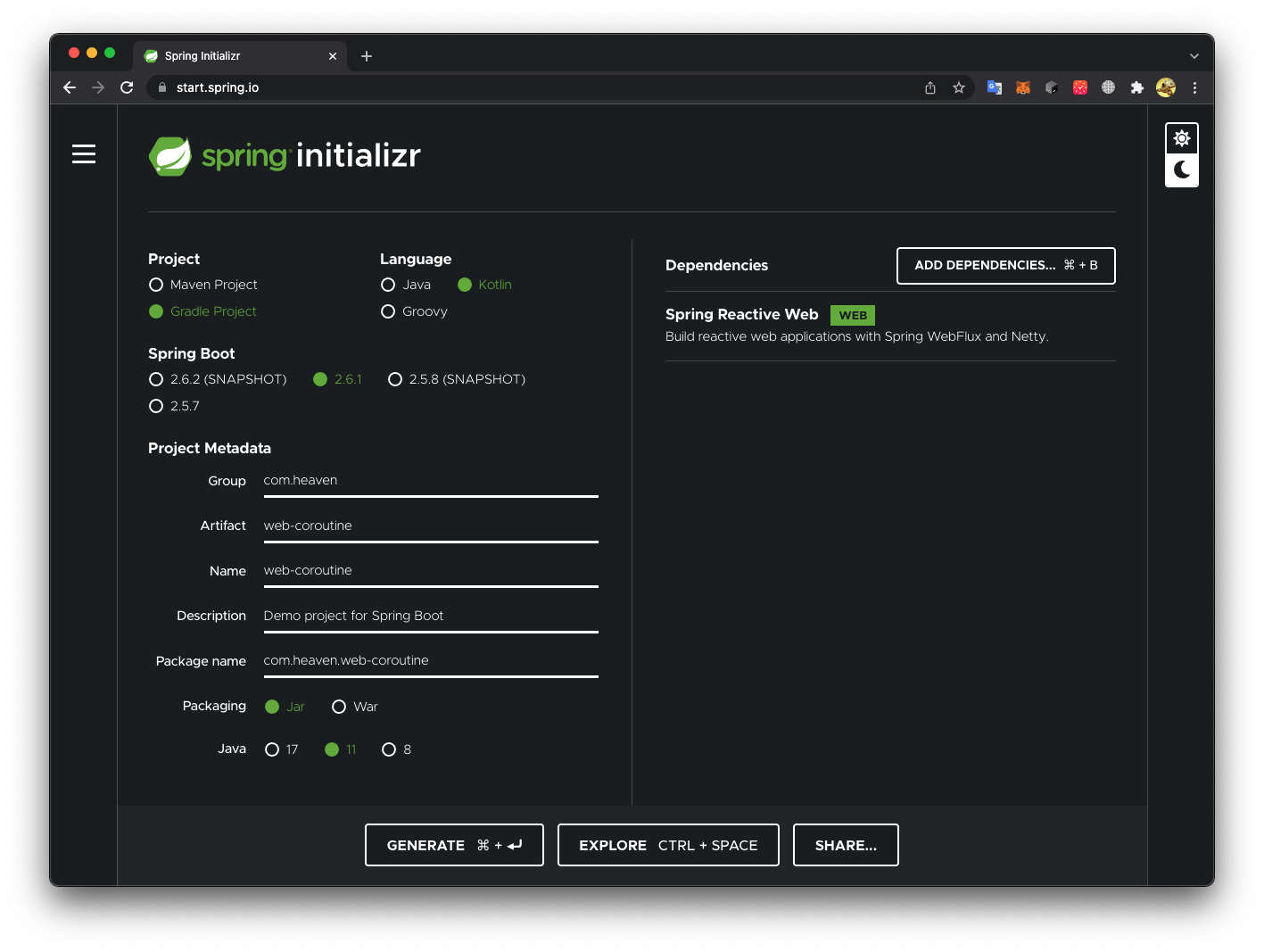
Figure 1. https://start.spring.io/
- 파일 정리
-
- HELP.md 파일 삭제
- src/main/resources/application.properties 의 확장자를 yml 로변경
1.2. Main 코드 작성
Hexagonal Architecture(Ports and Adapters Architecture) 를 내가 이해한데로 막 적용해 보자.
src/main/kotlin/com/haven/webcoroutine 아래 board 패키지 추가
1.2.1. Domain 구현
src/main/kotlin/com/haven/webcoroutine/board 아래 domain 패키지 추가
1.2.2. Entity 구현
src/main/kotlin/com/haven/webcoroutine/board/domain 아래 entity 패키지 추가
Board 클래스를 추가하고 아래와 같이 작성
Board.kt
|
|
1.2.3. Router 구현
src/main/kotlin/com/haven/webcoroutine/board/ 아래 outbound 패키지 추가
src/main/kotlin/com/haven/webcoroutine/board/outbound 아래 web 패키지 추가
BoardRouter 클래스를 추가하고 아래와 같이 작성
BoardRouter.kt
|
|
1.4. Test 코드 작성
1.4.1. Test 편하게 편하게… 의존성 추가
- kotest 라이브러리 추가
kotest 가 궁금하다면 남경호님의 글을 보자.
(P.S: Junit, Spock 따위…)
build.gradle.kts 에 의존성 추가
|
|
1.4.2. Test 구현
BoardRouterTest.kt
|
|
Test 를 실행해 보자.
웹 서버 그런 거 띄울 필요없다.
함수를 정의했고, 함수를 테스트하는데 굳이 웹 서버를 왜 띄워야 하는가?
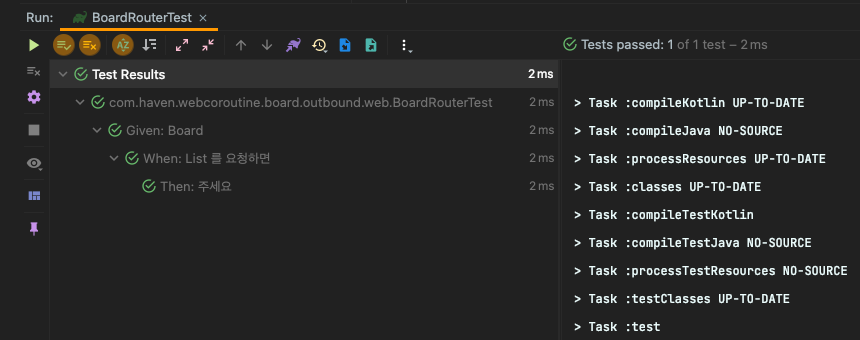